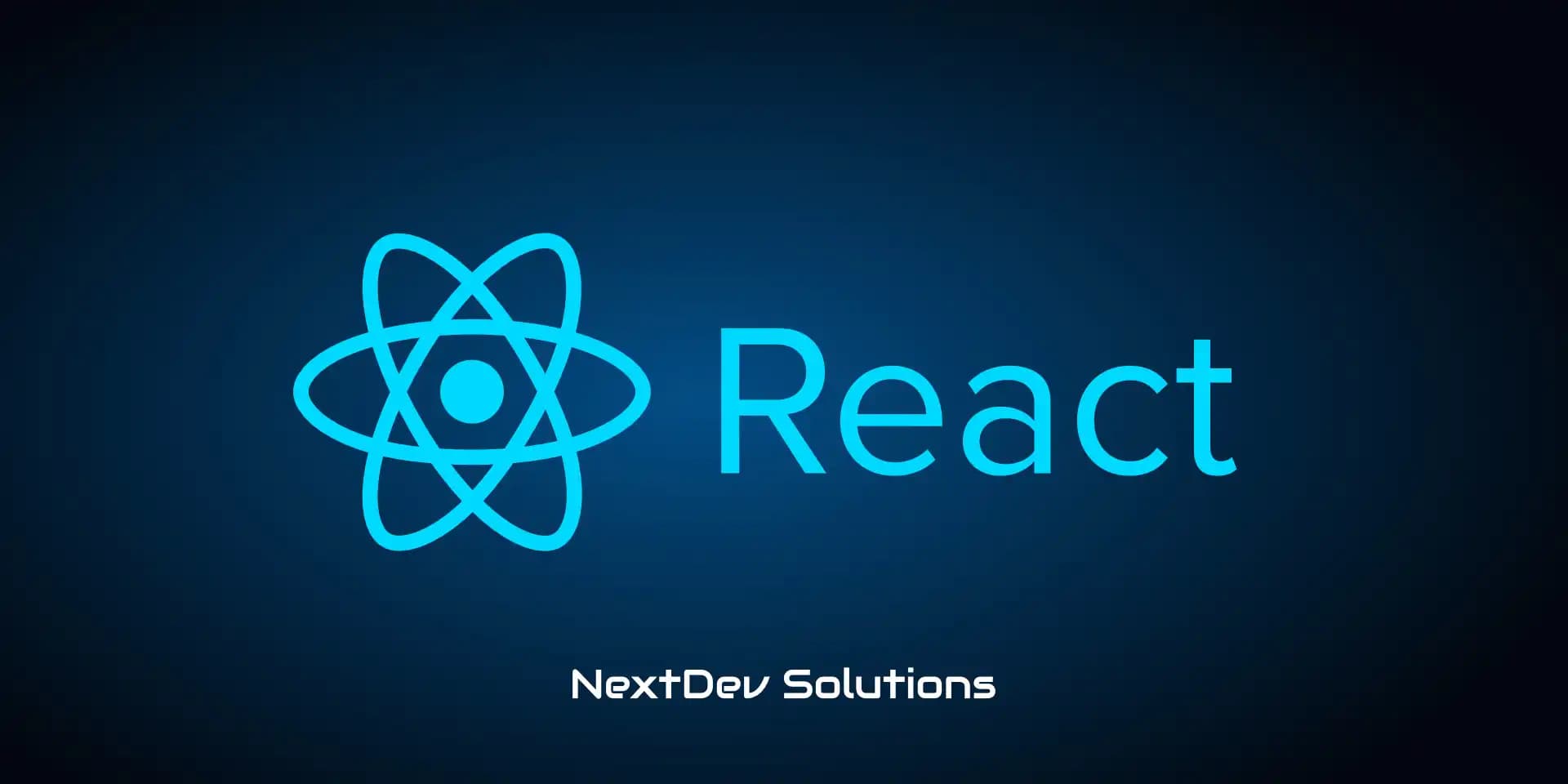
React: Building Dynamic and Interactive Interfaces
React is a powerful JavaScript library developed by Facebook in 2011. It has revolutionized the way developers build web applications. It allows for the creation of dynamic and interactive user interfaces by breaking down a webpage into small, reusable components. This modular approach to development helps in writing better-organized and maintainable code. Let's explore what React is, how to create a React application, and understand how React works under the hood.
What is React?
React is a JavaScript library designed for building dynamic and interactive user interfaces. It allows developers to describe a webpage using small, reusable components, making the development process more efficient and the codebase more manageable. Components are the building blocks of a React application, enabling developers to create modular, reusable, and better-organized code.
Creating a React Application
There are two popular ways to create a React application:
- Create React App (CRA): A widely-used tool that sets up a modern web application by running a single command.
- Vite: A newer build tool that offers a faster and leaner development experience.
Here’s how you can create a React app using Vite:
Create a new React app using Vite:
1npm create vite@latest 2cd react-app 3npm i 4code . 5npm run dev
Creating a React Component
In React, we create components using either JavaScript or TypeScript. Here’s an example of a simple React component in TypeScript:
Message Component (HelloReact)
1// PascalCasing for component names 2function HelloReact() { 3 // JSX syntax 4 const name = 'React'; 5 if (name) return <h1>Hello {name}</h1>; 6 return <h1>Hello World</h1>; 7} 8 9export default HelloReact;
Main Application Component (App)
1import HelloReact from "./HelloReact"; 2 3function App() { 4 return <div><HelloReact /></div>; 5} 6 7export default App;
In the HelloReact component, we use JSX (JavaScript XML) to define the UI structure. JSX allows us to write HTML-like syntax directly within JavaScript, making it easier to visualize the structure of our components
How React Works
A basic React application relies on two main libraries: react and react-dom.
- React: The core library for building user interfaces.
- ReactDOM: The package that handles rendering React components to the DOM.
When a React application starts, React builds a data structure in memory called the Virtual DOM. The Virtual DOM is a lightweight representation of the actual DOM and is used to keep track of changes in the component tree. Each node in the Virtual DOM represents a component.
When the state or data of a component changes, React updates the corresponding node in the Virtual DOM. It then compares the current version of the Virtual DOM with the previous version to identify the nodes that have changed. This process, known as reconciliation, ensures that only the necessary parts of the actual DOM are updated, resulting in efficient rendering and improved performance.
React Ecosystem
React is often compared to frameworks like Angular and Vue, but it's important to note the distinction:
- Library: A tool that provides specific functionality (e.g., React for UI components).
- Framework: A comprehensive set of tools and guidelines for building applications (e.g., Angular Vue and NextJS). React’s ecosystem includes various libraries and tools that complement its functionality, such as:
- UI Libraries: For building user interfaces (e.g., Material-UI, Ant Design).
- Routing: For handling navigation within the app (e.g., React Router).
- HTTP Requests: For making network requests (e.g., Axios, Fetch).
- State Management: For managing application state (e.g., Redux, Recoil).
- Internationalization: For supporting multiple languages (e.g., React Intl).
- Form Validation: For handling form validation (e.g., Formik, React Hook Form).
- Animations: For adding animations to the UI (e.g., React Spring, Framer Motion).
Conclusion
React provides a powerful and efficient way to build dynamic and interactive user interfaces. By leveraging components, developers can create reusable and modular code, leading to better-organized applications. With its robust ecosystem and strong community support, React continues to be a popular choice for front-end development. Whether you're a beginner or an experienced developer, React offers the tools and flexibility to build high-quality web applications.